Authenticating users
By default, Fernand's widget doesn't know the identity of the users interacting in widget. If you are dealing with logged in users, you can pass the "user" parameter to authenticate them.
Fernand('set', {
user: {
name: 'Richard',
email: 'richard@piedpiper.com'
}
})
In this scenario, whenever the user opens a new chat session, they won't be asked to provide their email or their name. They will be automatically identified, and all their previous conversations linked to Fernand, either via chat or email, listed in the Messages view.
To prevent bad actors from impersonating your users and passing any email to our widget through Javascript, we rely on the HMAC+SHA256 algorithm. You can sign and hash the user email, and pass it as the hash
parameter using the secret key available in your Fernand's dashboard.
When receiving the hash, we'll hash the email you provided with the same key and compare the resulting hash with the one you provided. If they match, we'll consider the email valid and verified.
Finding your secret key
You can find your secret key in Fernand's dashboard, under Settings → Channels, and then scrolling down to the Support widget section.
Click on "Show advanced settings", and you'll see the key to use for securing the emails under the "Authenticating users" section.
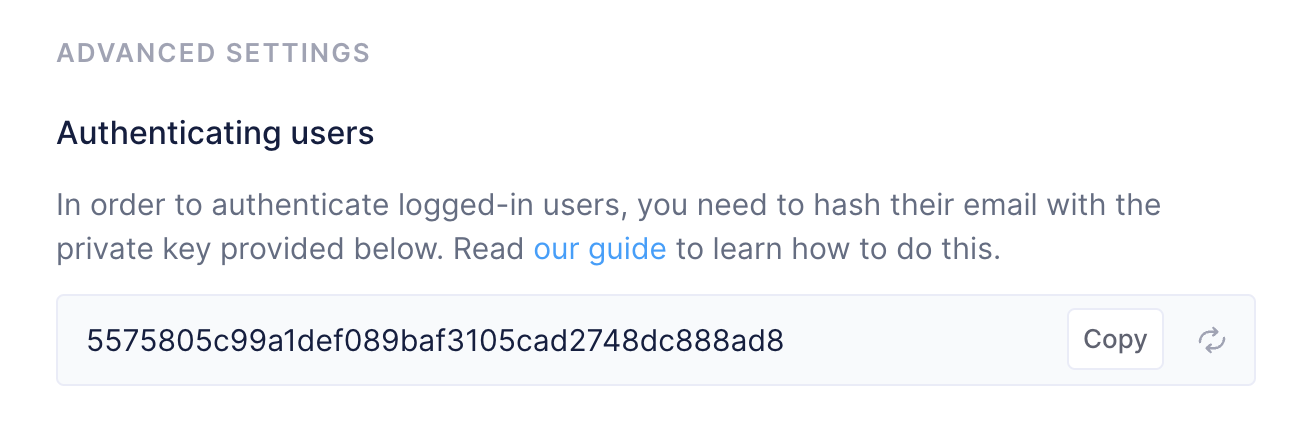
Hashing an email
To hash an email, you will need to use the HMAC+SHA256 mechanism.
We provide an example in Python, which is easily implemented, but you can do the same in all major programming languages.
In Python
import hmac, hashlib
hmac_digest = hmac.new(
key='6fdc0085d97cc621edcc0333fd93ef220f0eb1ce'.encode(),
msg='richard@piedpiper.com'.encode(),
digestmod=hashlib.sha256
).hexdigest()
print(hmac_digest) # fc3cf30c76a8b5c1c875b79c53a36bd8e2d653a1ec00c11fff538583ef759244
In Javascript
export const encode_hmac_sha256 = async (secret:string, message:string) => {
let enc = newTextEncoder()
let algorithm = { name: 'HMAC', hash:'SHA-256' }
let key = await crypto.subtle.importKey('raw', enc.encode(secret), algorithm, false, ['sign', 'verify'])
let signature = await crypto.subtle.sign(algorithm.name, key, enc.encode(message))
const hashArray = Array.from(newUint8Array(signature))
const digest = hashArray.map((b) => b.toString(16).padStart(2, '0')).join('')
return digest
}
Then, you can add this hash into the user's object like the following code example:
Fernand('set', {
user: {
name: 'Richard',
email: 'richard@piedpiper.com',
hash: 'fc3cf30c76a8b5c1c875b79c53a36bd8e2d653a1ec00c11fff538583ef759244'
}
})
Be careful that the hash is case sensitive. If you hash with an email having uppercase letters, but set the "email
" property in lower case, the hashes won't match.
Need help setting this up?
We appreciate this is a bit technical and could be a source of questions. If anything is unclear, please feel free to reach out at support@improvmx.com or.. on the widget in the bottom right, and we'll be right with you 🙂