Setting up the support widget
In addition to our email channels, you can set up a support widget on your app, product, website or any property that you include custom JS.
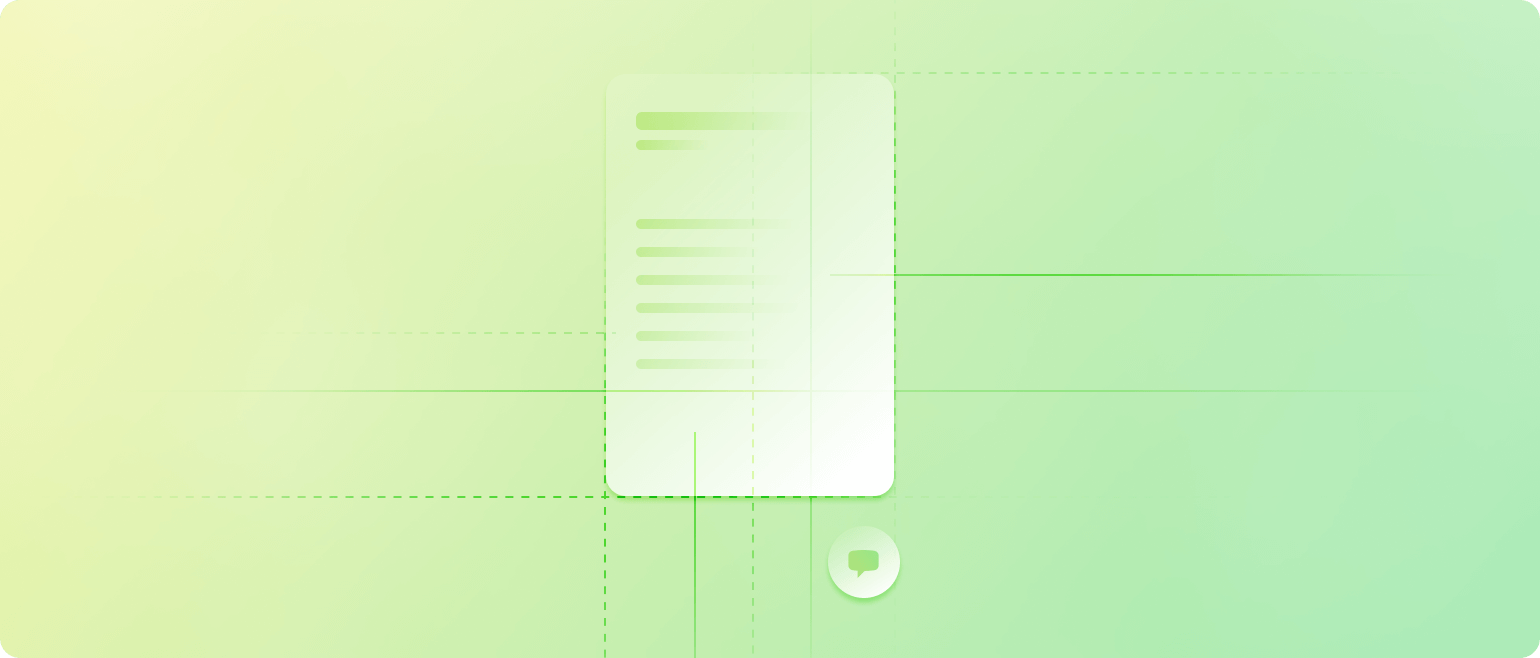
Our support widget lets your visitors or users find their way through your most important links via a customizable, embedded support widget showing on the bottom right of your page. It also let them contact you, either via a live chat or contact form interface, and let them fully browse your knowledge base content without ever leaving your product.
To embed our support widget on your site, you need to add this Javascript function to all the pages you want it to be shown on. We recommend to embed this in the <body> tag towards the end of your page, where your footer code lives, for example.
<script>
(function (w){if (typeof w.Fernand !== "function") {var f = function () {f.q[arguments[0] == 'set' ? 'unshift' : 'push'](arguments);};f.q = [];w.Fernand = f;}})(window);
Fernand('init', { appId: 'your-organization-name' })
</script>
<script async src="https://messenger.getfernand.com/client.js"></script>
Let's unpack what's happening here:
The first line of code setup the Fernand
function that you will be able to call to command the widget and its parameters.
The second line initialize the widget with one required parameter, appId
. The appId is the name of your organization used inside Fernand. You can find it in your URL when logging in on Fernand (e.g. https://app.getfernand.com/piedpiper/bonjour → your appId
is piedpiper
)
You will initiate the widget with Fernand('init', { appId: 'piedpiper' });
Settings
You can customize the widget by passing or updating a few parameters. You can do this by calling the set
method.
When you call Fernand('init', {...})
at the beginning, the second parameter is passed to Fernand('set', {...})
.
So the following:
Fernand('init', { appId: 'piedpiper', show: true, open: false })
Is a shortcut for the same as
Fernand('set', { appId: 'piedpiper', show: true, open: false })
Fernand('init')
Authenticating users
By default, Fernand's widget doesn't know the identity of the users interacting in widget. If you are dealing with logged in users, you can pass the "user" parameter to authenticate them.
Fernand('set', {
user: {
name: 'Richard',
email: 'richard@piedpiper.com'
}
})
We recommend that you add a verification hash to make sure that malicious actors don't impersonate your users. Learn more on authenticating logged in users.
List of settings
We support a wide array of options and settings to make the widget more personalized, and infused with your brand guideline.
appId
String — Required
This is the name of organization at Fernand, as indicated in the URL.show
Boolean — Optional, defaults totrue
Will define if the widget is visible at the bottom right, or hiddenopen
Boolean — defaults tofalse
If set to true, will open the widget automatically on page load. Note that if the user opens the widget and refresh the page, the widget will still memorize its state and re-open automatically.orgName
String
By default, our widget will display your organization's name as the widget's title, but you can override it by setting this value to anything you prefer. This is particularly helpful if you don't want to expose your legal entity name to your clients (e.g. PiedPiper LLC → Pied Piper)orgDescription
String
By default, this value is set to "Hey! How can we help?" and is displayed right under your organization name when opening the widget, but you can customize it too.sound
Boolean — defaults totrue
Emits a sound when the user receives a new message. You can disable it if you prefer.theme
String — defaults tonull
Define which color theme to use, between "dark" and "light." If you leave it undefined, the widget will use the user's system preferences.£tabs
Array — defaults to['home', 'chat', 'articles']
You can set which tabs are active on the widget. Possible values:home
Displays the homepage of the widget with your custom linkschat
Conversation experience with a chat UXarticles
Let users browse your knowledge basecontact
A basic contact form
defaultTab
String — defaults tohome
You can force the display of a tab other than 'home', like "Chat" or "Articles," by default when the user opens the widget.position
String — defaults toright
By default, the widget will be displayed on the right, but you can set the "position" to the following other values:
left
Displays the widget on the left side of the viewport
behavior
String — defaults todefault
default
Display the widget as a popup on top of the icon.modal
Displays the widget in the center as a modal. You can also set thehideIcon
property tofalse
if you want to implement your own custom launcher.sidepanel
Displays the widget as a panel on the right side of the page. If you also passposition
toleft
, it will display the panel on the left side of the page.
customPrompt
String — defaults to'Hi, how can we help?'
When the user creates a new conversation, you can choose which custom message prompt it displays. For instance, we recommend something like "We are happy to help! Reporting a bug? Please consider recording reproduction steps using https://jam.dev - it helps us a ton!".customIdentPrompt
String — defaults to'Thank you! We got your message but feel free to add anything'
This is the message that will displayed after the user has provided their email and name. We avoid repeating the same message ascustomPrompt
to avoid giving the feeling to the user that their original message wasn't saved.links
Array
This is an array of custom links that will be visible in the home tab. You can link to your status page, roadmap, calendar booking app, etc...hideIcon
Boolean — defaults tofalse
When set to true, the icon that shows the chat widget won't be displayed. It can be useful to set to true if you only want to open the widget on custom actions for instance.labels
Object
Allows you to change the label of each sections in the footer of the widget. Here are their keys and their default values:home
: "Home"chat
: "Chat"articles
: "Articles"contact
: "Contact Us"
This allows you to update the labels of any sections, for example:labels: { home: 'Welcome', articles: 'Knowledge' }
tags
Object
Allows you to define which tags to apply on new conversations for both the keys "chat
" and "contact
". Value for each keys (chat, contact) is an Array of string that are the related tags you want to apply.
Note that only existing tags will be applied. New ones will be ignored.
Example:tags: {chat: ['Chat', 'Support'], contact: ['Contact Form']}
Links structure
The links
parameter is an array of objects that contain four properties:
icon
String — defaults to 'link'
A predefined icon name from our set. We provide a few icons that you can choose from.title
String
The text displayed for the linkurl
String
The URL where to redirect the user to. If the URL hostname is the same as where the widget is, the page will open in the same tab, except if thetarget
property is set to "_blank".target
String
By defaults, lets the widget select what action to take on redirect: If the hostname of the redirect is the same as the current page, the redirect is made in that same page. Otherwise, or iftarget
is set to_blank
, a new tab is opened.
For the url
parameter, you can also set the following values to manipulate internal linking within the widget:
@chat
will open the Chat tab@article
will open the Articles tab@article:slug-of-an-article
will open a specific article in the Articles tab.
Functions
Once the widget has been initiated, you can trigger some actions by calling Fernand(...)
. Here are the list of actions you can do:
Fernand('init', {...})
Initialize the widget. This can only be done once.Fernand('set', {...})
Set the settings for the widget.Fernand('show')
Will show the widget's iconFernand('hide')
Will hide the widget's icon. If the widget is also open, it will close it first.Fernand('open')
Opens the widget in full. You can pass a second parameter which is the name of the tab you want to open (defaults to "home"), and a third in case of "articles", that is the slug of the article you want to open.Fernand('close')
Closes the widgetFernand('toggle')
Toggle between opening/closing the widgetFernand('destroy')
Destroy the widget, removing all HTML data and events listeners.Fernand('on', eventName, callback, once)
Add an event listener to events triggered by Fernand's widget, and will call thecallback
. You can pass the booleanonce
to trigger it one time or everytime.Fernand('off', eventName, callback)
Removes the event listener added previously
List of events you can listen to
Here's a list of events that will be triggered depending on the activity of the widget:
show
When the "show" event is triggeredshown
When the widget is fully shownhide
When the "hide" event is triggeredhidden
When the widget is fully hiddenopen
When the "open" event is triggeredopened
When the widget is fully openclose
When the "close" event is triggeredclosed
When the widget is fully closedmessage
When a new message is receiveddestroyed
When the widget is destroyed