Table
The Table component allows you to display structured data in rows and columns. Tables are especially useful for displaying sets of data like users, domains, or statuses in an organized way.
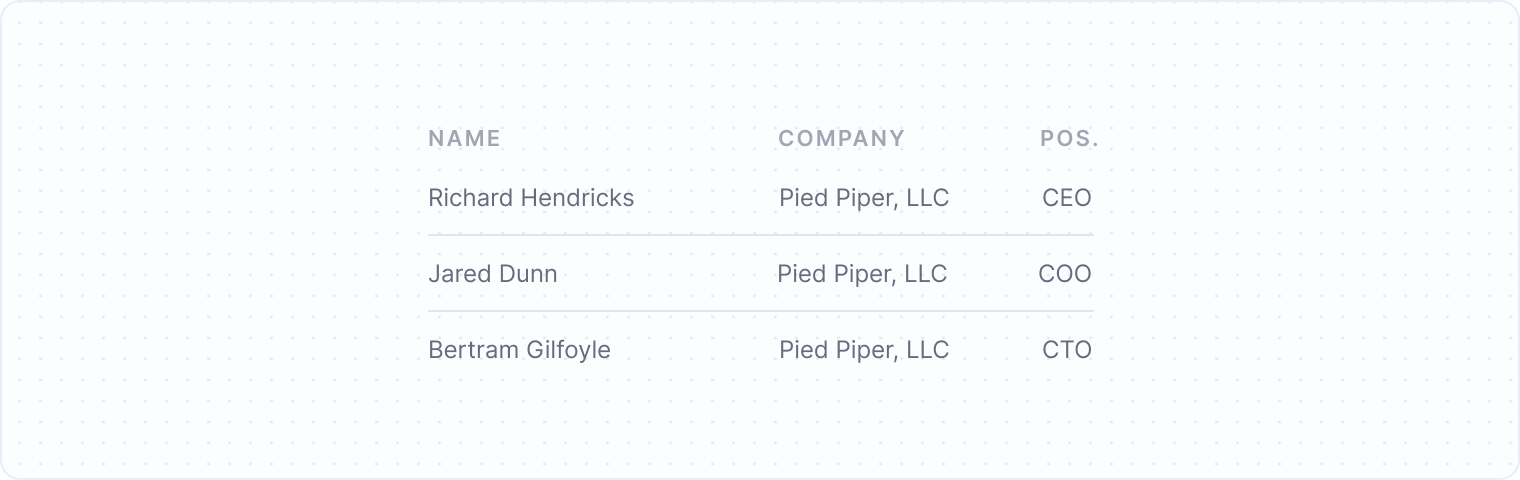
Basic table
To create a table in Fernand, use the standard HTML <table>
element wrapped inside a <div class="table__control">
. Here’s a simple table structure with a header and some rows:
<div class="table__control">
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Status</th>
</tr>
</thead>
<tbody>
<tr>
<td>Alice</td>
<td>alice@example.com</td>
<td>Active</td>
</tr>
<tr>
<td>Bob</td>
<td>bob@example.com</td>
<td>Inactive</td>
</tr>
</tbody>
</table>
</div>
This will generate a simple table displaying user information, neatly contained within the table__control
class.
Using tables with Liquid
In Fernand, you can leverage Liquid to dynamically populate your tables with data retrieved from APIs. Here’s an example that loops through a list of users and populates the table:
<div class="table__control">
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Status</th>
</tr>
</thead>
<tbody>
{% for user in users.get('items') %}
<tr>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
<td>
<span class="pill {% if user.active == true %}pill--green{% else %}pill--red{% endif %}">
{% if user.active == true %}Active{% else %}Inactive{% endif %}
</span>
</td>
</tr>
{% endfor %}
</tbody>
</table>
</div>
This example uses a for each loop to iterate over a list of users. For each user, it displays their name, email, and status. Conditional logic is applied to show the status with a green badge for active users and a red badge for inactive users.
Handling empty tables
Sometimes, your data set may be empty. To handle this, you can use conditional statements in Liquid to display a message if no data is available:
<div class="table__control">
<table>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Status</th>
</tr>
</thead>
<tbody>
{% if users.get('items') | size == 0 %}
<tr>
<td colspan="3">No users found.</td>
</tr>
{% else %}
{% for user in users.get('items') %}
<tr>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
<td>
<span class="{% if user.active == true %}badge--green{% else %}badge--red{% endif %}">
{% if user.active == true %}Active{% else %}Inactive{% endif %}
</span>
</td>
</tr>
{% endfor %}
{% endif %}
</tbody>
</table>
</div>
This example checks if there are any users before displaying the table. If no users are found, it shows a message saying, "No users found."